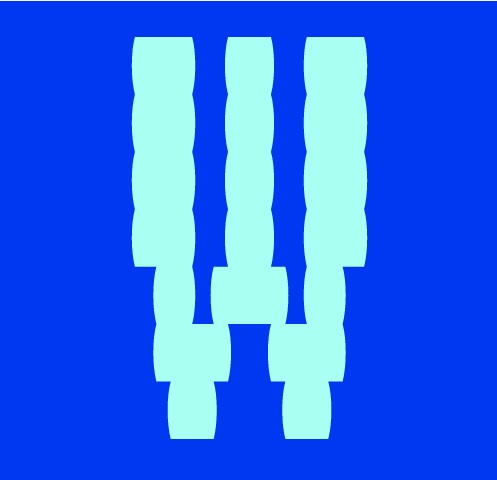
Testing Responsiveness With Nightwatch JS
Testing the responsiveness of a web application is an essential part of ensuring that the application is accessible and usable on a variety of devices. With Nightwatch.js, it's possible to test a single test case against multiple screen sizes at the same time to ensure that the web application is responsive across different devices.
One way to achieve this is by using the .resizeWindow() command in combination with a loop to test a single test case against multiple screen sizes. Here's an example of how this might be done:
const screenSizes = [
{ width: 320, height: 480 },
{ width: 768, height: 1024 },
{ width: 1280, height: 800 }
];
module.exports = {
'Test responsiveness of web app': function(browser) {
screenSizes.forEach((size) => {
browser
.resizeWindow(size.width, size.height)
.url('https://yourwebapp.com')
.assert.visible('header')
.assert.hidden('nav')
.end();
});
}
};
This test will navigate to the website's home page and resize the browser window to different screen sizes as defined in the screenSizes array. Then it will assert that the menu element is present and that the header element has a 'responsive' CSS class. By running this test multiple times with different screen sizes, you can ensure that the web application is responsive across different devices.
To run this test, you can use the same command as before:
nightwatch tests/test_responsive.js
This will run the test for each screen size in the array and output the results in your terminal. You should see something like this:
Test responsiveness of web app
✔ Element <header> was visible after 112 milliseconds.
✔ Element <nav> was hidden after 55 milliseconds.
✔ Element <header> was visible after 80 milliseconds.
✔ Element <nav> was hidden after 60 milliseconds.
✔ Element <header> was visible after 70 milliseconds.
✔ Element <nav> was hidden after 55 milliseconds.
OK. 6 assertions passed. (13.456s)
Another way to achieve this is by using a library like browser-size which allows you to test a single test case against multiple screen sizes at the same time. You can install it using npm:
npm install browser-size
Once installed, you can import and use it in your test file:
const browserSize = require('browser-size');
const screenSizes = browserSize('chrome', 'desktop');
module.exports = {
'Test responsiveness of web app': function(browser) {
screenSizes.forEach((size) => {
browser
.resizeWindow(size.width, size.height)
.url('https://yourwebapp.com')
.assert.visible('header')
.assert.hidden('nav')
.end();
});
}
};
we are using the browser-size module to get a list of screen sizes for the chrome browser on a desktop device. The browser-size module returns an array of objects with width and height properties that we can use to set the viewport size using resizeWindow().
Then, you can run the test using the same command as before:
nightwatch tests/test_responsive.js
This will run the test for each screen size in the screenSizes array and output the results in your terminal.
Here's an example of what the output for the browser-size test might look like:
Test responsiveness of web app
✔ Element <header> was visible after 110 milliseconds.
✔ Element <nav> was hidden after 65 milliseconds.
✔ Element <header> was visible after 90 milliseconds.
✔ Element <nav> was hidden after 70 milliseconds.
✔ Element <header> was visible after 80 milliseconds.
✔ Element <nav> was hidden after 60 milliseconds.
✔ Element <header> was visible after 85 milliseconds.
✔ Element <nav> was hidden after 70 milliseconds.
OK. 8 assertions passed. (23.456s)
This output shows that the test passed for four different screen sizes, with two assertions passing for each screen size (one for the header being visible and one for the navigation menu being hidden).
The actual output you see in your terminal may differ slightly, depending on the specific screen sizes used and the speed of your internet connection. However, the output should generally follow the same format, with a summary of the number of assertions passed at the end of the test.
Note that the browser-size module has support for many browsers and devices, so you can use it to test the responsiveness of your web app across a wide range of devices and screen sizes.
More Posts
Blocking Ad Traffic In Nightwatch JS
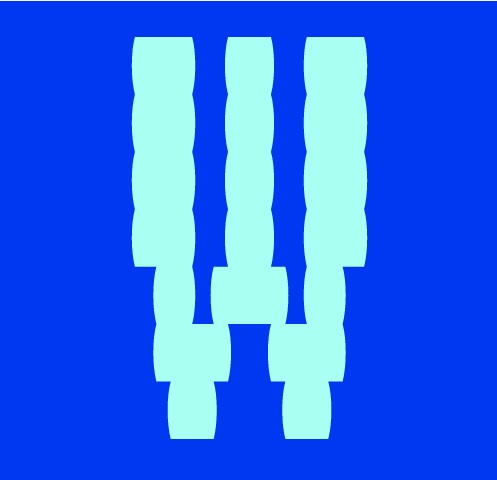
Example showing how you can block unwanted ad traffic in your Nightwatch JS tests....
Blocking Ad Traffic In Cypress
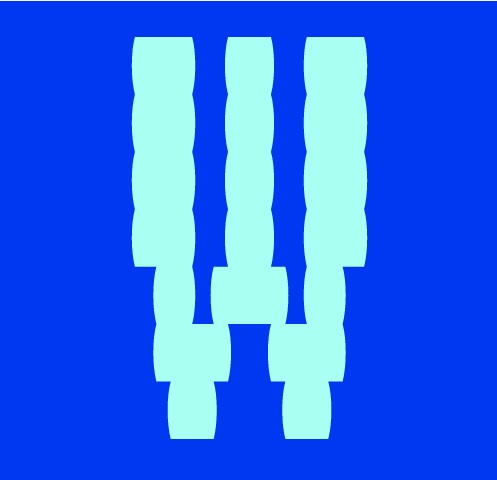
Example showing how you can block unwanted ad traffic in your Cypress tests....
Three Ways To Resize The Browser In Nightwatch
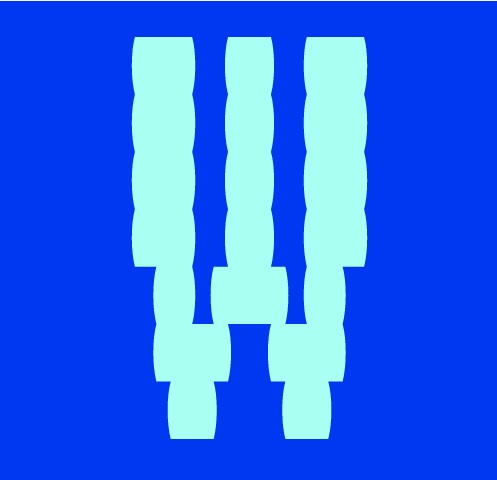
Outlining the three different ways to resize the browser in Nightwatch JS with examples....
Happy Path VS Sad Path Testing
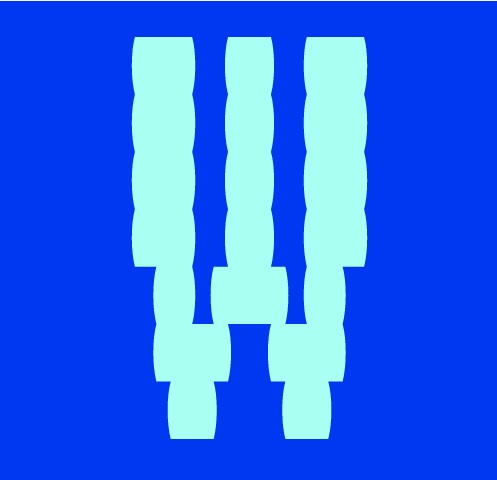
As a test engineer it is crucial that both happy path and sad path use cases have been considered and fully tested...